Python Linked List: Delete the last item from a singly linked list
Python Linked List: Exercise-7 with Solution
Write a Python program to delete the last item from a singly linked list.
Sample Solution:-
Python Code:
class Node:
# Singly linked node
def __init__(self, data=None):
self.data = data
self.next = None
class singly_linked_list:
def __init__(self):
# Createe an empty list
self.tail = None
self.head = None
self.count = 0
def append_item(self, data):
#Append items on the list
node = Node(data)
if self.head:
self.head.next = node
self.head = node
else:
self.tail = node
self.head = node
self.count += 1
def delete_item(self, data):
# Delete an item from the list
current = self.tail
prev = self.tail
while current:
if current.data == data:
if current == self.tail:
self.tail = current.next
else:
prev.next = current.next
self.count -= 1
return
prev = current
current = current.next
def iterate_item(self):
# Iterate the list.
current_item = self.tail
while current_item:
val = current_item.data
current_item = current_item.next
yield val
items = singly_linked_list()
items.append_item('PHP')
items.append_item('Python')
items.append_item('C#')
items.append_item('C++')
items.append_item('Java')
print("Original list:")
for val in items.iterate_item():
print(val)
print("\nAfter removing the last item from the list:")
items.delete_item('Java')
for val in items.iterate_item():
print(val)
Sample Output:
Original list: PHP Python C# C++ Java After removing the last item from the list: PHP Python C# C++
Flowchart:
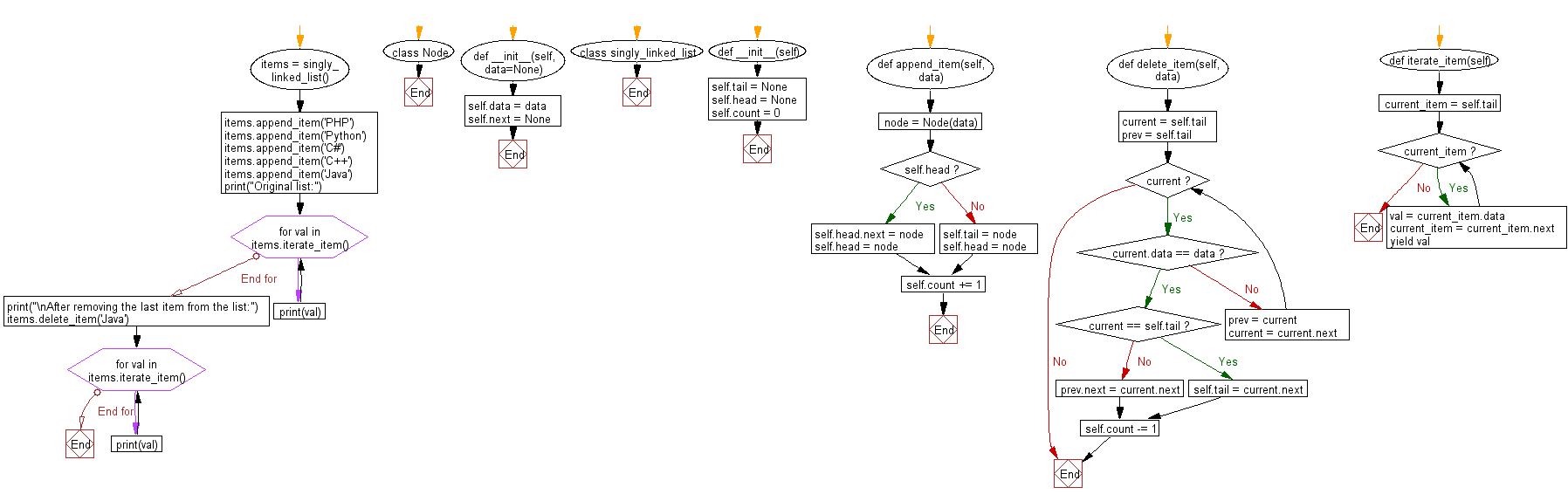
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to delete the first item from a singly linked list.
Next: Write a Python program to create a doubly linked list, append some items and iterate through the list (print forward).
What is the difficulty level of this exercise?
Test your Python skills with w3resource's quiz
Python: Tips of the Day
Creates a dictionary with the same keys as the provided dictionary and values generated by running the provided function for each value:
Example:
def tips_map_values(obj, fn): ret = {} for key in obj.keys(): ret[key] = fn(obj[key]) return ret users = { 'Owen': { 'user': 'Owen', 'age': 29 }, 'Eddie': { 'user': 'Eddie', 'age': 15 } } print(tips_map_values(users, lambda u : u['age'])) # {'Owen': 29, 'Eddie': 15}
Output:
{'Owen': 29, 'Eddie': 15}
- New Content published on w3resource:
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- React - JavaScript Library
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework