Python Linked List: Print a given doubly linked list in reverse order
Python Linked List: Exercise-11 with Solution
Write a Python program to print a given doubly linked list in reverse order.
Sample Solution:-
Python Code:
class Node(object):
# Singly linked node
def __init__(self, data=None, next=None, prev=None):
self.data = data
self.next = next
self.prev = prev
class doubly_linked_list(object):
def __init__(self):
self.head = None
self.tail = None
self.count = 0
def append_item(self, data):
# Append an item
new_item = Node(data, None, None)
if self.head is None:
self.head = new_item
self.tail = self.head
else:
new_item.prev = self.tail
self.tail.next = new_item
self.tail = new_item
self.count += 1
def iter(self):
# Iterate the list
current = self.head
while current:
item_val = current.data
current = current.next
yield item_val
def print_foward(self):
for node in self.iter():
print(node)
def reverse(self):
""" Reverse linked list. """
current = self.head
while current:
temp = current.next
current.next = current.prev
current.prev = temp
current = current.prev
temp = self.head
self.head = self.tail
self.tail = temp
items = doubly_linked_list()
items.append_item('PHP')
items.append_item('Python')
items.append_item('C#')
items.append_item('C++')
items.append_item('Java')
items.append_item('SQL')
print("Reverse list ")
items.reverse()
items.print_foward()
Sample Output:
Reverse list SQL Java C++ C# Python PHP
Flowchart:
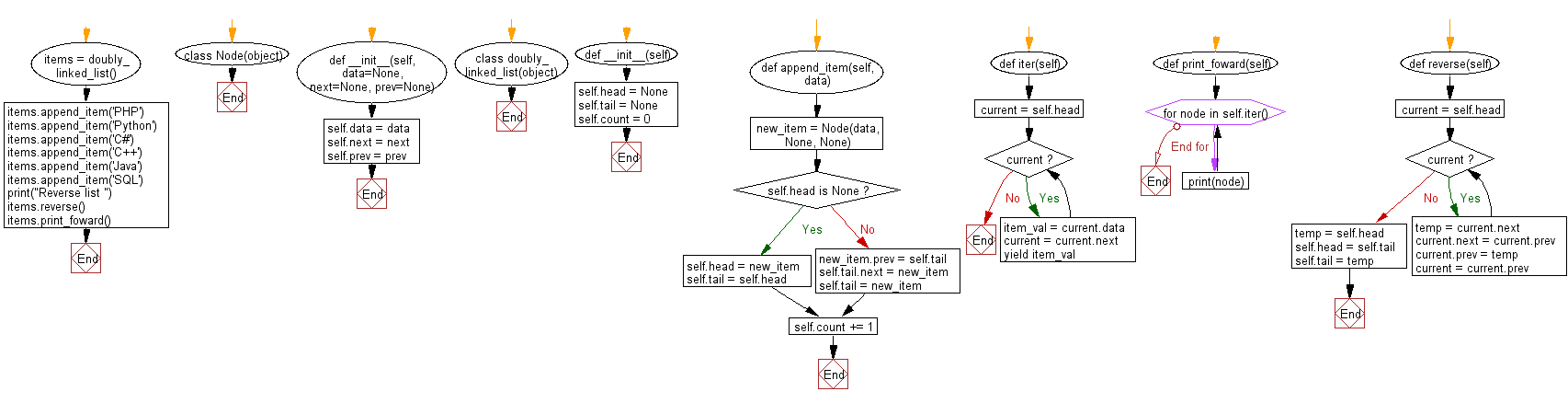
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to count the number of items of a given doubly linked list.
Next: Write a Python program to insert an item in front of a given doubly linked list.
What is the difficulty level of this exercise?
Test your Python skills with w3resource's quiz
Python: Tips of the Day
Creates a dictionary with the same keys as the provided dictionary and values generated by running the provided function for each value:
Example:
def tips_map_values(obj, fn): ret = {} for key in obj.keys(): ret[key] = fn(obj[key]) return ret users = { 'Owen': { 'user': 'Owen', 'age': 29 }, 'Eddie': { 'user': 'Eddie', 'age': 15 } } print(tips_map_values(users, lambda u : u['age'])) # {'Owen': 29, 'Eddie': 15}
Output:
{'Owen': 29, 'Eddie': 15}
- New Content published on w3resource:
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- React - JavaScript Library
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework