Python: Find the class wise roll number from a tuple-of-tuples
Python Collections: Exercise-32 with Solution
Write a Python program to find the class wise roll number from a tuple-of-tuples.
Sample Solution:
Python Code:
from collections import defaultdict
classes = (
('V', 1),
('VI', 1),
('V', 2),
('VI', 2),
('VI', 3),
('VII', 1),
)
class_rollno = defaultdict(list)
for class_name, roll_id in classes:
class_rollno[class_name].append(roll_id)
print(class_rollno)
Sample Output:
defaultdict(<class 'list'>, {'V': [1, 2], 'VI': [1, 2, 3], 'VII': [1]})
Flowchart:
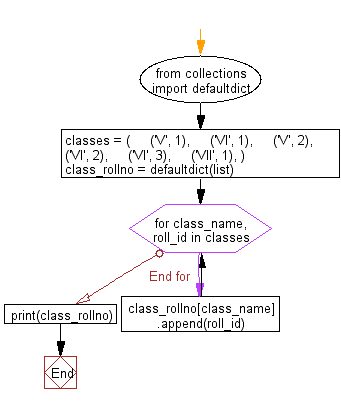
Visualize Python code execution:
The following tool visualize what the computer is doing step-by-step as it executes the said program:
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to count the most common words in a dictionary.
Next: Write a Python program to count the number of students of individual class.
What is the difficulty level of this exercise?
Test your Python skills with w3resource's quiz
Python: Tips of the Day
Use Enumerate() In for Loops:
>>> students = ('John', 'Mary', 'Mike') >>> for i, student in enumerate(students): ... print(f'Iteration: {i}, Student: {student}') ... Iteration: 0, Student: John Iteration: 1, Student: Mary Iteration: 2, Student: Mike >>> for i, student in enumerate(students, 35001): ... print(f'Student Name: {student}, Student ID #: {i}') ... Student Name: John, Student ID #: 35001 Student Name: Mary, Student ID #: 35002 Student Name: Mike, Student ID #: 35003
- New Content published on w3resource:
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- React - JavaScript Library
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework