Python: Frequency of the elements in a given list of lists using collections module
Python Collections: Exercise-29 with Solution
Write a Python program to get the frequency of the elements in a given list of lists. Use collections module.
Sample Solution:
Python Code:
from collections import Counter
from itertools import chain
nums = [
[1,2,3,2],
[4,5,6,2],
[7,1,9,5],
]
print("Original list of lists:")
print(nums)
print("\nFrequency of the elements in the said list of lists:")
result = Counter(chain.from_iterable(nums))
print(result)
Sample Output:
Original list of lists: [[1, 2, 3, 2], [4, 5, 6, 2], [7, 1, 9, 5]] Frequency of the elements in the said list of lists: Counter({2: 3, 1: 2, 5: 2, 3: 1, 4: 1, 6: 1, 7: 1, 9: 1})
Flowchart:
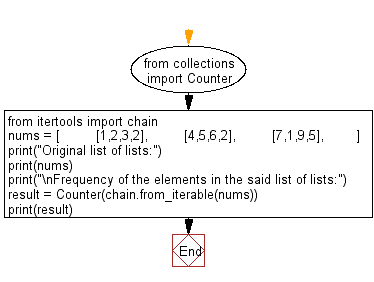
Visualize Python code execution:
The following tool visualize what the computer is doing step-by-step as it executes the said program:
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to create a dictionary grouping a sequence of key-value pairs into a dictionary of lists. Use collections module.
Next: Write a Python program to count the occurrence of each element of a given list.
What is the difficulty level of this exercise?
Test your Python skills with w3resource's quiz
Python: Tips of the Day
Given a predicate function, fn, and a prop string, this curried function will then take an object to inspect by calling the property and passing it to the predicate:
Example:
def tips_check_prop(fn, prop): return lambda obj: fn(obj[prop]) check_age = tips_check_prop(lambda x: x >= 25, 'age') user = {'name': 'Owen', 'age': 25} print(check_age(user))
Output:
True
- New Content published on w3resource:
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- React - JavaScript Library
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework