Python: Find the characters which occur in more than and less than a given number in list of strings
Python Collections: Exercise-25 with Solution
Write a Python program to find the characters in a list of strings which occur more than and less than a given number.
Sample Solution:
Python Code:
from collections import Counter
from itertools import chain
def max_aggregate(list_str, N):
temp = (set(sub) for sub in list_str)
counts = Counter(chain.from_iterable(temp))
gt_N = [chr for chr, count in counts.items() if count > N]
lt_N = [chr for chr, count in counts.items() if count < N]
return gt_N, lt_N
list_str = ['abcd', 'iabhef', 'dsalsdf', 'sdfsas', 'jlkdfgd']
print("Original list:")
print(list_str)
N = 3
result = max_aggregate(list_str, N)
print("\nCharacters of the said list of strings which occur more than:",N)
print(result[0])
print("\nCharacters of the said list of strings which occur less than:",N)
print(result[1])
Sample Output:
Original list: ['abcd', 'iabhef', 'dsalsdf', 'sdfsas', 'jlkdfgd'] Characters of the said list of strings which occur more than: 3 ['a', 'd', 'f'] Characters of the said list of strings which occur less than: 3 ['c', 'b', 'h', 'e', 'i', 's', 'l', 'k', 'j', 'g']
Flowchart:
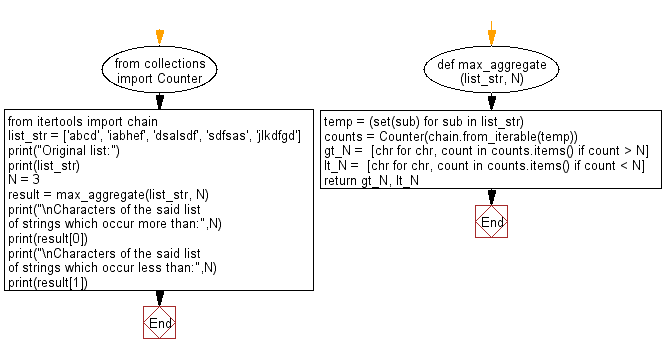
Visualize Python code execution:
The following tool visualize what the computer is doing step-by-step as it executes the said program:
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to calculate the maximum aggregate from the list of tuples (pairs).
Next: Write a Python program to find the difference between two list including duplicate elements. Use collections module.
What is the difficulty level of this exercise?
Test your Python skills with w3resource's quiz
Python: Tips of the Day
Inverts a dictionary with unique hashable values:
Example:
def tips_invert_dictionary(obj): return { value: key for key, value in obj.items() } ages = { "Owen": 29, "Eddie": 15, "Jhon": 22, } print(tips_invert_dictionary(ages))
Output:
{29: 'Owen', 15: 'Eddie', 22: 'Jhon'}
- New Content published on w3resource:
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- React - JavaScript Library
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework