Python: Counter arithmetic and set operations
Python Collections: Exercise-16 with Solution
Write a Python program to perform Counter arithmetic and set operations for aggregating results.
Sample Solution:
Python Code:
import collections
c1 = collections.Counter([1, 2, 3, 4, 5])
c2 = collections.Counter([4, 5, 6, 7, 8])
print('C1:', c1)
print('C2:', c2)
print('\nCombined counts:')
print(c1 + c2)
print('\nSubtraction:')
print(c1 - c2)
print('\nIntersection (taking positive minimums):')
print(c1 & c2)
print('\nUnion (taking maximums):')
print(c1 | c2)
Sample Output:
C1: Counter({1: 1, 2: 1, 3: 1, 4: 1, 5: 1}) C2: Counter({4: 1, 5: 1, 6: 1, 7: 1, 8: 1}) Combined counts: Counter({4: 2, 5: 2, 1: 1, 2: 1, 3: 1, 6: 1, 7: 1, 8: 1}) Subtraction: Counter({1: 1, 2: 1, 3: 1}) Intersection (taking positive minimums): Counter({4: 1, 5: 1}) Union (taking maximums): Counter({1: 1, 2: 1, 3: 1, 4: 1, 5: 1, 6: 1, 7: 1, 8: 1})
Flowchart:
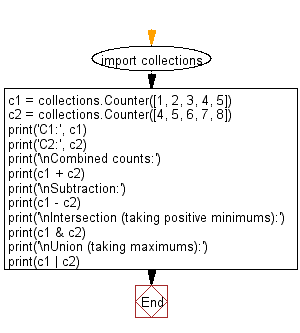
Visualize Python code execution:
The following tool visualize what the computer is doing step-by-step as it executes the said program:
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the most common element of a given list.
Next: Write a Python program to find the majority element from a given array of size n using Collections module.
What is the difficulty level of this exercise?
Test your Python skills with w3resource's quiz
Python: Tips of the Day
Creates a dictionary with the same keys as the provided dictionary and values generated by running the provided function for each value:
Example:
def tips_map_values(obj, fn): ret = {} for key in obj.keys(): ret[key] = fn(obj[key]) return ret users = { 'Owen': { 'user': 'Owen', 'age': 29 }, 'Eddie': { 'user': 'Eddie', 'age': 15 } } print(tips_map_values(users, lambda u : u['age'])) # {'Owen': 29, 'Eddie': 15}
Output:
{'Owen': 29, 'Eddie': 15}
- New Content published on w3resource:
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- React - JavaScript Library
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework