C Exercises: Find the largest palindrome made from the product of two 3-digit numbers
C Programming Practice: Exercise-21 with Solution
2520 is the smallest number that can be divided by each of the numbers from 1 to 10 without any remainder.
Write a C programming to find the smallest positive number that is evenly divisible by all of the numbers from 1 to 20?.
C Code:
/* Copyright (c) 2009, eagletmt, Released under the MIT License <http://opensource.org/licenses/mit-license.php> */
#include <stdio.h>
static unsigned long gcd(unsigned long a, unsigned long b);
static __inline unsigned long lcm(unsigned long a, unsigned long b);
int main(void)
{
unsigned long ans = 1;
unsigned long i;
for (i = 1; i <= 20; i++) {
ans = lcm(ans, i);
}
printf("%lu\n", ans);
return 0;
}
unsigned long gcd(unsigned long a, unsigned long b)
{
unsigned long r;
if (a > b) {
unsigned long t = a;
a = b;
b = t;
}
while (r = a%b) {
a = b;
b = r;
}
return b;
}
unsigned long lcm(unsigned long a, unsigned long b)
{
unsigned long long p = (unsigned long long)a * b;
return p/gcd(a, b);
}
Sample Output:
232792560
Flowchart:
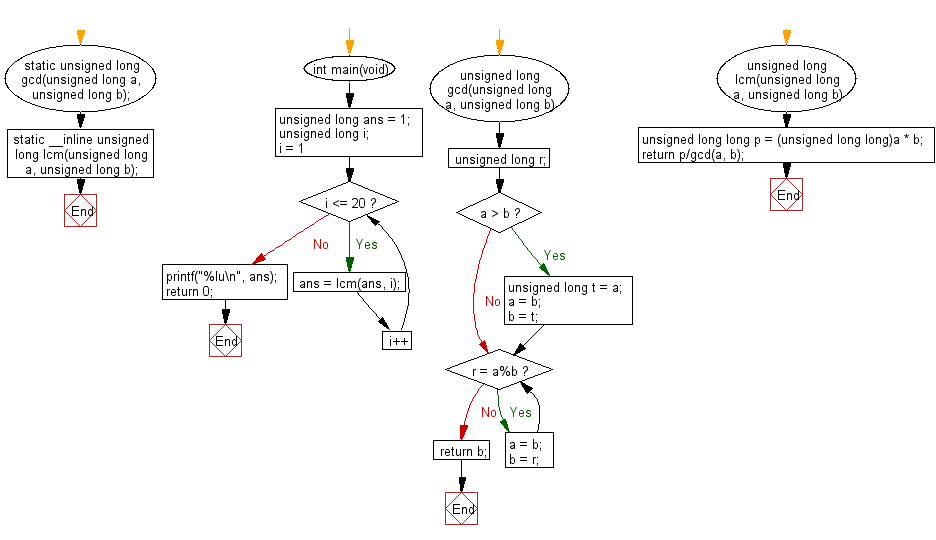
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C programming to find the largest palindrome made from the product of two 3-digit numbers.
Next: Write a C programming to find the difference between the sum of the squares of the first one hundred natural numbers and the square of the sum.
What is the difficulty level of this exercise?
C Programming: Tips of the Day
C Programming - Why do all the C files written by my lecturer start with a single # on the first line?
In the very early days of pre-standardised C, if you wanted to invoke the preprocessor, then you had to write a # as the first thing in the first line of a source file. Writing only a # at the top of the file affords flexibility in the placement of the other preprocessor directives.
From an original C draft by the great Dennis Ritchie himself:
12. Compiler control lines
[...] In order to cause [the] preprocessor to be invoked, it is necessary that the very first line of the program begin with #. Since null lines are ignored by the preprocessor, this line need contain no other information.
That document makes for great reading (and allowed me to jump on this question like a mad cat).
I suspect it's the lecturer simply being sentimental - it hasn't been required certainly since ANSI C.
Ref : https://bit.ly/2Mb8OVZ
- New Content published on w3resource:
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- React - JavaScript Library
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework