Python JSON: Convert JSON encoded data into Python objects
Python JSON: Exercise-5 with Solution
Write a Python program to convert JSON encoded data into Python objects.
Sample Solution:-
Python Code:
import json
jobj_dict = '{"name": "David", "age": 6, "class": "I"}'
jobj_list = '["Red", "Green", "Black"]'
jobj_string ='"Python Json"'
jobj_int ='1234'
jobj_float = '21.34'
python_dict = json.loads(jobj_dict)
python_list = json.loads(jobj_list)
python_str = json.loads(jobj_string)
python_int = json.loads(jobj_int)
python_float = json.loads(jobj_float)
print("Python dictionary: ", python_dict)
print("Python list: ", python_list)
print("Python string: ", python_str)
print("Python integer: ", python_int)
print("Python float: ", python_float)
Output:
Python dictionary: {'name': 'David', 'age': 6, 'class': 'I'} Python list: ['Red', 'Green', 'Black'] Python string: Python Json Python integer: 1234 Python float: 21.34
Flowchart:
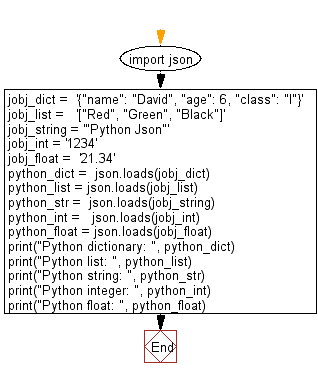
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to convert Python dictionary object (sort by key) to JSON data. Print the object members with indent level 4.
Next: Write a Python program to create a new JSON file from an existing JSON file.
What is the difficulty level of this exercise?
Test your Python skills with w3resource's quiz
Python: Tips of the Day
Python: Unknown Arguments Using *arguments
If your function can take in any number of arguments then add a * in front of the parameter name:
def myfunc(*arguments): for a in arguments: print a myfunc(a) myfunc(a,b) myfunc(a,b,c)
- New Content published on w3resource :
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- React - JavaScript Library
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework