Проекты Python: Magic 8 Ball для гадания или поиска совета
Python Project-5 с решением
Создайте на Python проект Magic 8 Ball, представляющий собой игрушку для гадания или получения совета.
Замечания :
- Разрешить пользователю вводить свой вопрос.
- Показать текущее сообщение.
- Создайте 10/20 ответов и покажите случайный ответ.
- Разрешить пользователю задать другой вопрос / совет или выйти из игры.
Образец раствора -1:
Код Python:
#Make a Magic 8 ball
#https://github.com/viljow/magic8/blob/master/main.py
import random
answers = ['It is certain', 'It is decidedly so', 'Without a doubt', 'Yes – definitely', 'You may rely on it', 'As I see it, yes', 'Most likely', 'Outlook good', 'Yes Signs point to yes', 'Reply hazy', 'try again', 'Ask again later', 'Better not tell you now', 'Cannot predict now', 'Concentrate and ask again', 'Dont count on it', 'My reply is no', 'My sources say no', 'Outlook not so good', 'Very doubtful']
print(' __ __ _____ _____ _____ ___ ')
print(' | \/ | /\ / ____|_ _/ ____| / _ \ ')
print(' | \ / | / \ | | __ | || | | (_) |')
print(' | |\/| | / /\ \| | |_ | | || | > _ < ')
print(' | | | |/ ____ \ |__| |_| || |____ | (_) |')
print(' |_| |_/_/ \_\_____|_____\_____| \___/ ')
print('')
print('')
print('')
print('Hello World, I am the Magic 8 Ball, What is your name?')
name = input()
print('hello ' + name)
def Magic8Ball():
print('Ask me a question.')
input()
print (answers[random.randint(0, len(answers)-1)] )
print('I hope that helped!')
Replay()
def Replay():
print ('Do you have another question? [Y/N] ')
reply = input()
if reply =='Y':
Magic8Ball()
elif reply =='N':
exit()
else:
print('I apologies, I did not catch that. Please repeat.')
Replay()
Magic8Ball()
Выход:
__ __ _____ _____ _____ ___ | // | // / ____ | _ _ / ____ | / _ / | / / | / / | | __ | || | | (_) | | | // | | / // / | | | _ | | || | > _ < | | | | / ____ / | __ | | _ | || | ____ | (_) | | _ | | _ / _ / / _ / _____ | _____ / _____ | / ___ / Привет мир, я Волшебный 8 бал, как тебя зовут? Сара привет Сара Задайте мне вопрос. Скажи мне удачу Это точно Я надеюсь, что это помогло! У вас есть еще один вопрос? [Y / N] Y Задайте мне вопрос. Мой любимый цвет Мой ответ нет Я надеюсь, что это помогло! У вас есть еще один вопрос? [Y / N] N
Блок - схема:
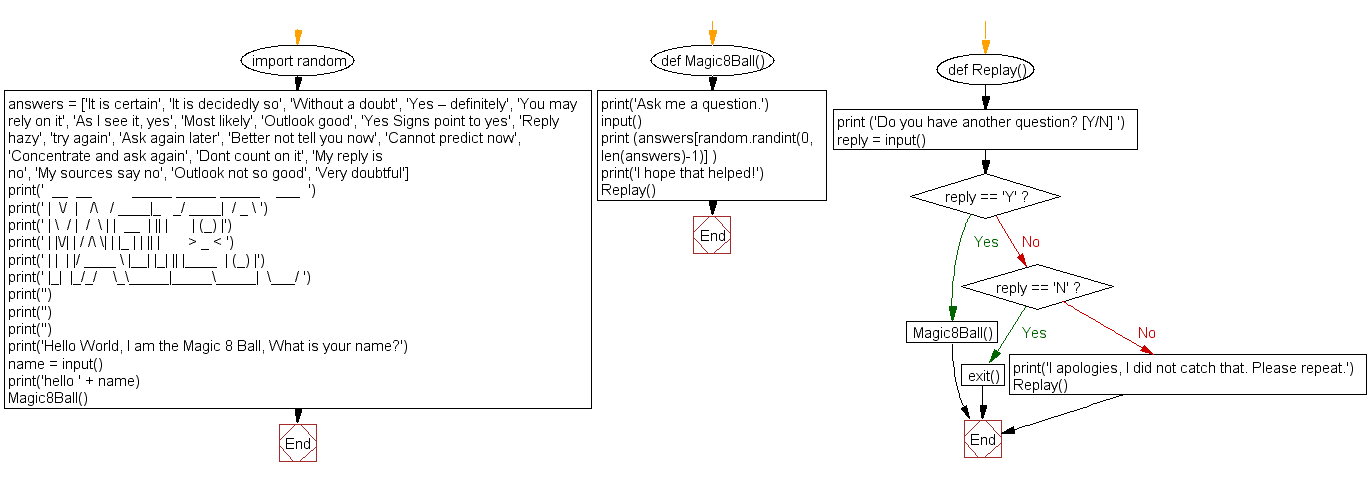
Образец раствора -2:
Код Python:
#https://github.com/soupyck/Magic8Ball/blob/master/magic8ball.py
import random
import time
eight_ball = [ "It is certain", "It is decidedly so", "Without a doubt", "Yes, definitely",
"You may rely on it", "As I see it, yes", "Most Likely", "Outlook Good",
"Yes", "Signs point to yes", "Reply hazy, try again", "Ask again later",
"Better not tell you now", "Cannot predict now", "Concentrate and ask again",
"Don't count on it", "My reply is no", "My sources say no", "Outlook not so good", "Very Doubtful"]
def question():
question = input("You may ask your yes or no question of the Magic 8 Ball!\n")
print("Thinking...")
time.sleep(random.randrange(0,5))
print(random.choice(eight_ball))
while True:
question()
repeat = input("Would you like to ask another question? (Y or N)")
if not (repeat =="y" or repeat =="Y"):
print("Come back if you have more questions!")
break
Пример вывода:
Вы можете задать свой вопрос да или нет о Magic 8 Ball! Скажите мне мое состояние Мышление ... Вы можете положиться на него Хотите задать еще один вопрос? (Y или N) Y Вы можете задать свой вопрос да или нет о Magic 8 Ball! да Мышление ... Определенно да Хотите задать еще один вопрос? (Y или N) n Вернись, если у вас есть еще вопросы!
Блок - схема:

Пример решения -3:
99 бутылок пива ...
Код Python:
https://github.com/pixelnull/8ball/blob/master/8ball.py
import random
import time
# Set count to how many times user wants to do the magic 8ball
count_str = input("Hello user. How many questions would you like to ask the 8-Ball? ")
# Handling if count_str is not a number
while True:
if count_str.isdigit():
count = int(count_str)
break
else:
count_str = input("You cheeky devil, just enter a simple number this time: ")
# Outlier input handling
if count <= 0:
print("Well I didn't want to play with you either!")
time.sleep(5)
exit()
elif count > 10:
print("\nYou have to be kidding me, you want to do that many questions?\nWe'll do 1 and go from there.\n", sep='')
count = 1
# Sequence for random.choice
answers = ["It is certain.", "It is decidedly so.", "Without a doubt", "Yes definitely.", "You may rely on it.",
"As I see it, yes.", "Most likely.", "Outlook good.", "Yes.", "Signs point to yes.", "Reply hazy try again.",
"Ask again later.", "Better not tell you now.", "Cannot predict now.", "Concentrate and ask again.",
"Don\'t count on it.", "My reply is no.", "My sources say no.", "Outlook not so good.", "Very doubtful."]
# Main loop and graceful exit
while count > 0:
blah = input("Type your question: ")
dice = random.choice(answers)
print("\n", dice, "\n", sep='')
time.sleep(2)
count = count - 1
else:
print("\nI hope you liked the answer(s). THE GREAT 8-BALL HAS SPOKEN!")
time.sleep(5)
exit()
Пример вывода:
Привет пользователь. Сколько вопросов вы хотели бы задать 8-Ball? 1 Введите свой вопрос: Скажите мне мое состояние Знаки указывают на да. Надеюсь, вам понравились ответы. БОЛЬШОЙ 8-МЯЧ РАЗГОВОРЕН!
Блок - схема:
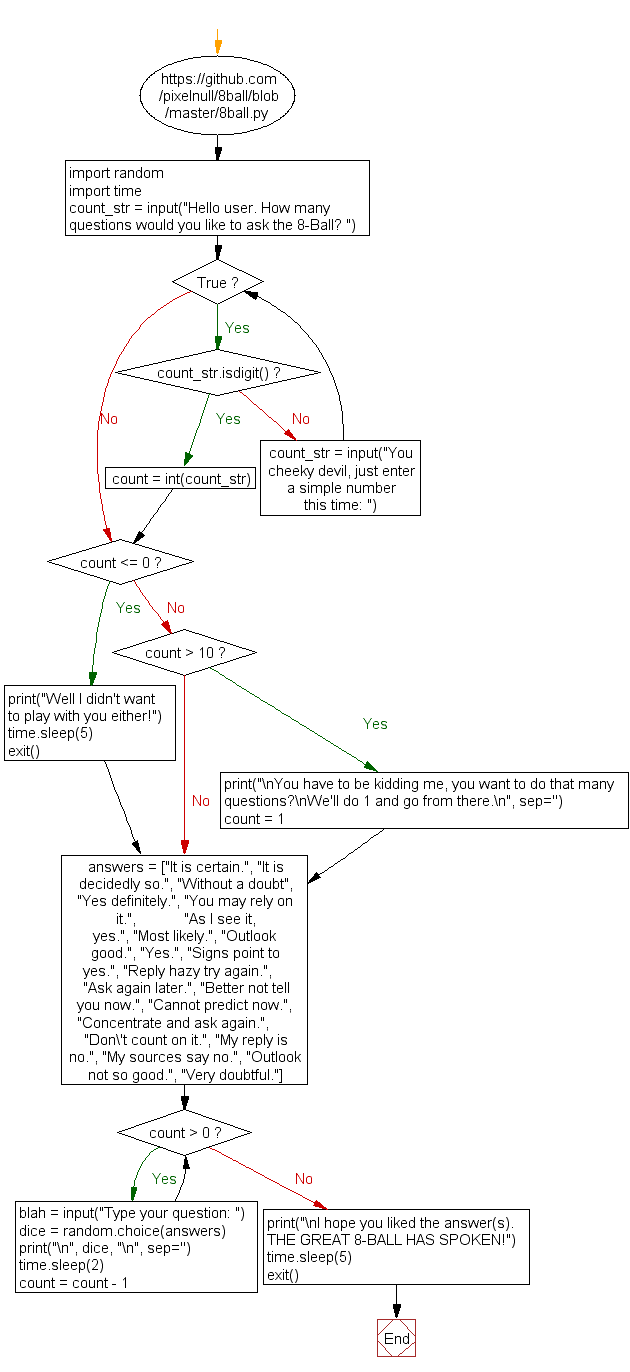
Пример решения -4:
Код Python:
#https://github.com/DeronKHolmes/magic-8-ball-game/blob/master/eightball.py
import random
import time, sys
play_again ='yes'
while play_again =='yes':
str(input("Welcome to the Magic 8-Ball. Enter your question: ")).lower()
#Delay output for 1 second each
print("Thinking...")
time.sleep(1)
print("3...")
time.sleep(1)
print("2...")
time.sleep(1)
print("1...")
time.sleep(1)
print()
#Generate a random integer/response
response = random.randint(0,20)
if response == 1:
print("Not just no, hell no!")
elif response == 2:
print("Sure thing.")
elif response == 3:
print("Don't count on it.")
elif response == 4:
print("Maybe not.")
elif response == 5:
print("Count on it.")
elif response == 6:
print("The Universe says maybe.")
elif response == 7:
print("I don't see why not.")
elif response == 8:
print("The future looks good for you.")
elif response == 9:
print("That's for sure.")
elif response == 10:
print("Maybe.")
elif response == 11:
print("There's a chance.")
elif response == 12:
print("Certainly!")
elif response == 13:
print("Keep doing what you're doing and it'll happen.")
elif response == 14:
print("Not over my dead 8 Ball.")
elif response == 15:
print("No.")
elif response == 16:
print("Yes.")
elif response == 17:
print("All depends on if you've been good for Santa this year.")
elif response == 18:
print("Not in this lifetime.")
elif response == 19:
print("Someday, but not today.")
elif response == 20:
print("Right after you hit the lottery.")
else:
print("Not a valid question!")
play_again = str(input("Would you like to ask another question? yes/no ")).lower()
if play_again =='no':
print("Goodbye! Thanks for playing!")
sys.exit()
Пример вывода:
Добро пожаловать в Волшебный 8-Ball. Введите свой вопрос: Скажите мне мое состояние Мышление ... 3 ... 2 ... 1 ... Возможно, нет. Хотите задать еще один вопрос? да / нет да Добро пожаловать в Волшебный 8-Ball. Введите свой вопрос: мой любимый цвет Мышление ... 3 ... 2 ... 1 ... Нет. Хотите задать еще один вопрос? да / нет нет До свидания! Спасибо за игру!
Блок - схема:
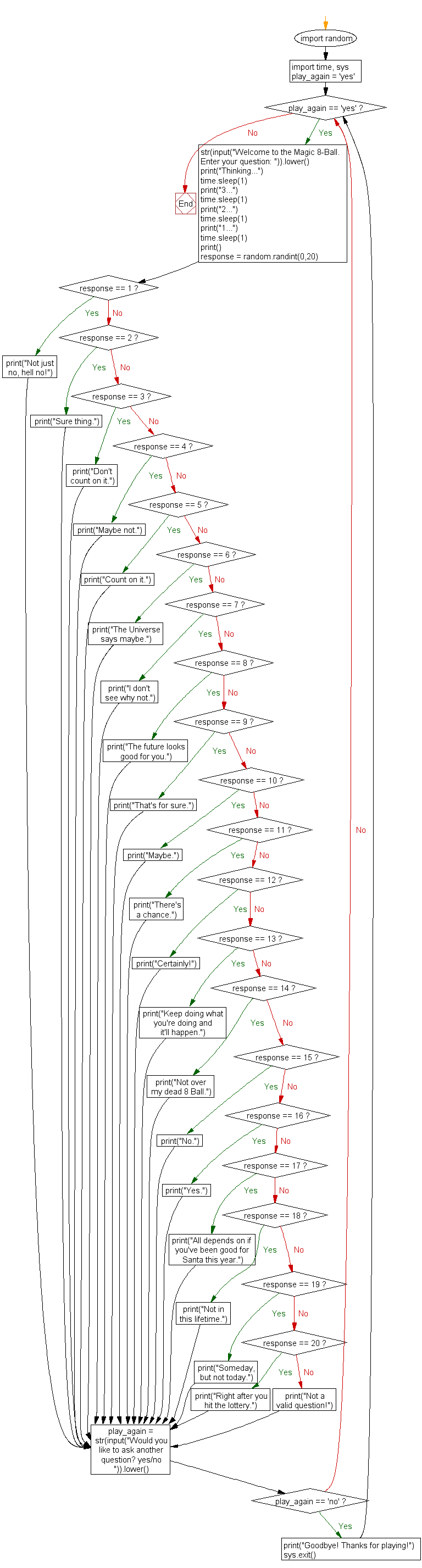
Внесите свой код и комментарии через Disqus.
Новый контент: Composer: менеджер зависимостей для PHP , R программирования
disqus2code