Проекты Python: Распечатайте песню 99 бутылок пива на стене
Python Project-4 с решением
Создайте проект Python, который распечатывает каждую строчку песни «99 бутылок пива на стене».
Примечание: попробуйте использовать встроенную функцию вместо того, чтобы вручную вводить все строки.
Образец раствора -1:
Код Python:
# https://github.com/luongthomas/Python-Mini-Projects/blob/master/99%20Bottles/99bottles.py
# Purpose: A program printing out every line to the song "99 bottles of beer on the wall"
# Notes: Besides the phrase "take one down", can't manually type numbers/names into song
# When reached 1 bottle left, "bottles" becomes singular
def sing(n):
if (n == 1):
objects ='bottle'
objectsMinusOne ='bottles'
elif (n == 2):
objects ='bottles'
objectsMinusOne ='bottle'
else:
objects ='bottles'
objectsMinusOne ='bottles'
if (n > 0):
print(str(n) + " " + objects + " of beer on the wall, " + str(n) + " " + objects + " of beer.")
print("Take one down and pass it around, " + str(n-1) + " " + objectsMinusOne + " of beer on the wall.")
print(" ")
elif (n == 0):
print("No more bottles of beer on the wall, no more bottles of beer.")
print("Go to the store and buy some more, 99 bottles of beer on the wall.")
else:
print("Error: Wheres the booze?")
bottles = 99
while bottles >= 0:
sing(bottles)
bottles -= 1
Выход:
99 бутылок пива на стене, 99 бутылок пива. Сними один и раздай, 98 бутылок пива на стене. 98 бутылок пива на стене, 98 бутылок пива. Снеси один и раздай, 97 бутылок пива на стене. .......................................... 63 бутылки пива на стене, 63 бутылки пива. Сними один и раздай, 62 бутылки пива на стене. 62 бутылки пива на стене, 62 бутылки пива. Сними один и раздай, 61 бутылка пива на стене. .......................................... 2 бутылки пива на стене, 2 бутылки пива. Снеси один и раздай его, 1 бутылка пива на стене. 1 бутылка пива на стене, 1 бутылка пива. Сними один и раздай, 0 бутылок пива на стене. Нет больше бутылок пива на стене, нет больше бутылок пива. Идите в магазин и купите еще 99 бутылок пива на стене.
Блок - схема:
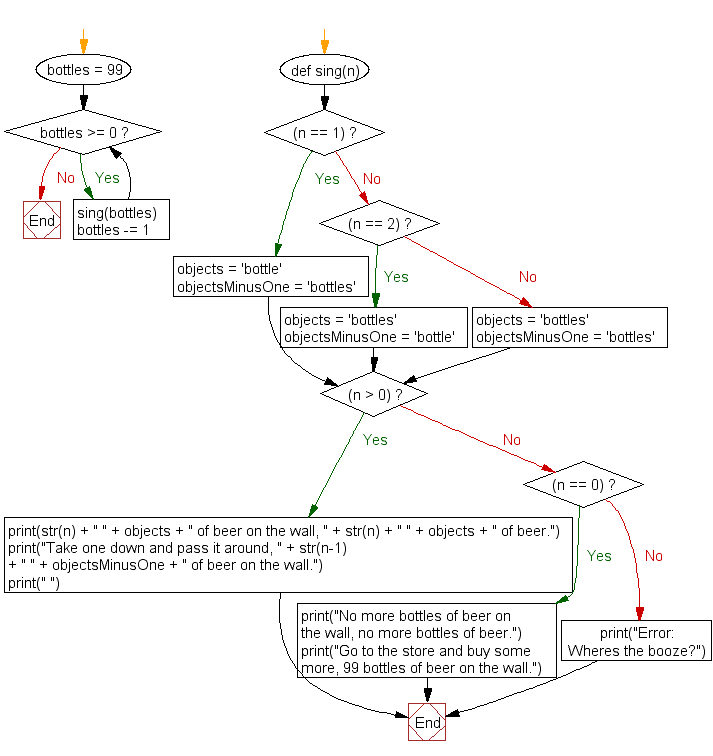
Образец раствора -2:
99 бутылок пива ...
Код Python:
import time
# https://github.com/marcgushwa/99bottles/blob/master/99.py
## 99 Bottles of beer on the wall
## 99 Bottles of beer
## Take one down, pass it around
## 98 bottles of beer on the wall
bottles = 99
while bottles > 0:
print(bottles,"bottles of beer on the wall")
print(bottles,"bottles of beer")
print("Take one down, pass it around")
bottles = bottles - 1
print(bottles,"bottles of beer on the wall")
time.sleep(1)
Пример вывода:
99 бутылок пива на стене 99 бутылок пива Сними один, раздай 98 бутылок пива на стене .............................. 62 бутылки пива на стене 62 бутылки пива ............................. 2 бутылки пива на стене 2 бутылки пива на стене 2 бутылки пива Сними один, раздай 1 бутылка пива на стене 1 бутылка пива на стене 1 бутылка пива Сними один, раздай 0 бутылок пива на стене
Блок - схема:
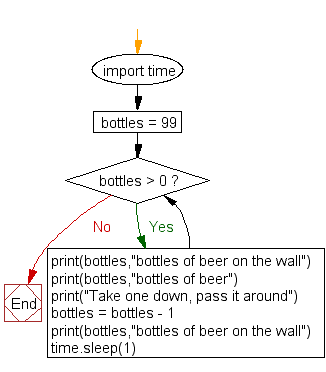
Пример решения -3:
99 бутылок пива ...
Код Python:
# https://github.com/RianGallagher/Beginner-projects-solutions/blob/master/99bottles.py
for i in range(99, 0, -1):
if(i == 2):
print("{} bottles of beer on the wall, {} bottles of beer, take one down, pass it around, {} bottle of beer on the wall".format(i, i, i-1))
elif(i == 1):
print("{} bottle of beer on the wall, {} bottle of beer, take one down, pass it around, no bottles of beer on the wall".format(i, i))
else:
print("{} bottles of beer on the wall, {} bottles of beer, take one down, pass it around, {} bottles of beer on the wall".format(i, i, i-1))
Пример вывода:
99 бутылок пива на стене, 99 бутылок пива, возьмите одну, раздайте, 98 бутылок пива на стене 98 бутылок пива на стене, 98 бутылок пива, возьмите одну, раздайте, 97 бутылок пива на стене ........................ 61 бутылка пива на стене, 61 бутылка пива, возьми одну, разнеси, 60 бутылок пива на стене ........................ 2 бутылки пива на стене, 2 бутылки пива, возьмите одну, раздайте, 1 бутылка пива на стене 1 бутылка пива на стене, 1 бутылка пива, возьмите одну, раздайте ее, никаких бутылок пива на стене
Блок - схема:
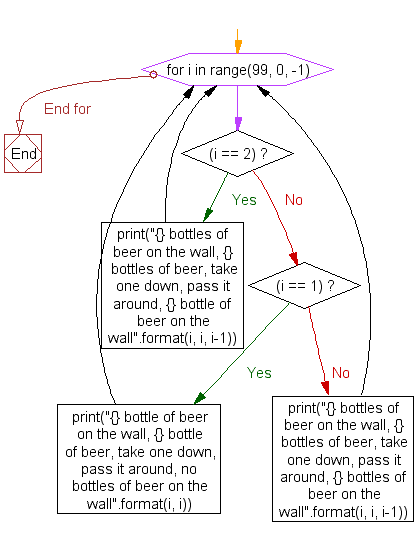
Пример решения -4:
99 бутылок пива ...
Код Python:
# https://github.com/Everstalk/BP/blob/master/99%20Bottles.py
def counter():
for i in range(99,-1,-1):
if i == 0:
print ("No more bottles of beer on the wall, no more bottles of beer.")
print ("Go to the store and buy some more,99 bottles of beer on the wall")
elif i == 1:
print ("1 bottle of beer on the wall, 1 bottle of beer.")
print ("Take one down and pass it around, no more bottles of beer to go")
elif i == 2:
print (i," bottles of beer on the wall, ",i," bottles of beer.")
print ("Take one down and pass it around, ",i-1," bottle of beer on the wall.")
else :
print (i," bottles of beer on the wall, ",i," bottles of beer.")
print ("Take one down and pass it around, ",i-1," bottles of beer on the wall.")
counter()
Пример вывода:
99 бутылок пива на стене, 99 бутылок пива. Сними один и раздай, 98 бутылок пива на стене. 98 бутылок пива на стене, 98 бутылок пива. ..................................... 61 бутылка пива на стене, 61 бутылка пива. Сними один и раздай, 60 бутылок пива на стене. 60 бутылок пива на стену, 60 бутылок пива. ..................................... 1 бутылка пива на стене, 1 бутылка пива. Сними один и раздай его, бутылок пива больше не будет Нет больше бутылок пива на стене, нет больше бутылок пива. Пойдите в магазин и купите еще, 99 бутылок пива на стене
Блок - схема:
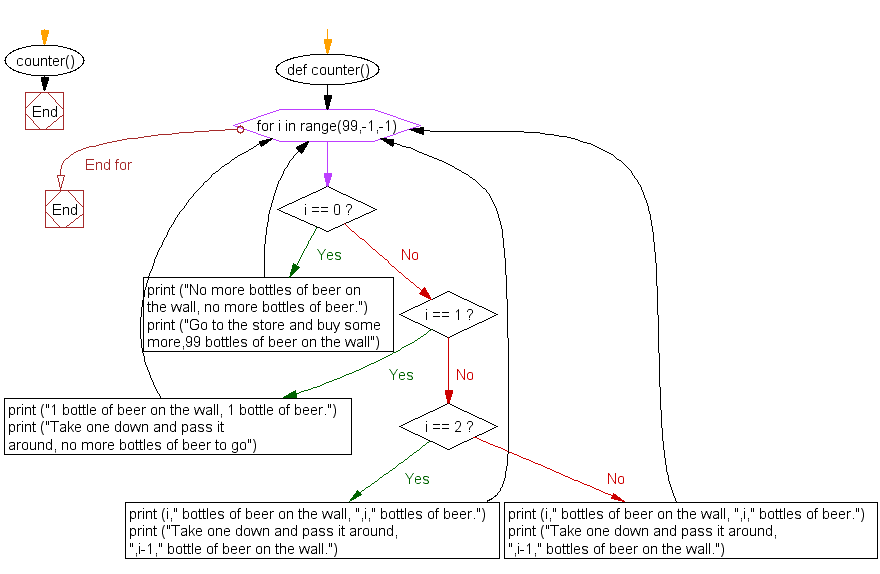
Улучшите этот пример решения и опубликуйте свой код через Disqus
Новый контент: Composer: менеджер зависимостей для PHP , R программирования
disqus2code