Проекты Python: Угадай число, которое выбрано случайным образом
Python Project-3 с решением
Создайте проект Python, чтобы угадать число, которое выбрано случайным образом.
Образец раствора -1:
Код Python:
#https://github.com/sarbajoy/NumberGuess/blob/master/numberguess.py
import random
number=random.randrange(0,100)
guessCheck="wrong"
print("Welcome to Number Guess")
while guessCheck=="wrong":
response=int(input("Please input a number between 0 and 100:"))
try:
val=int(response)
except ValueError:
print("This is not a valid integer. Please try again")
continue
val=int (response)
if val<number:
print("This is lower than actual number. Please try again.")
elif val>number:
print("This is higher than actual number. Please try again.")
else:
print("This is the correct number")
guessCheck="correct"
print("Thank you for playing Number Guess. See you again")
Пример вывода:
Добро пожаловать в номер думаю Пожалуйста, введите число от 0 до 100: 6 Это ниже, чем фактическое число. Пожалуйста, попробуйте еще раз. Пожалуйста, введите число от 0 до 100: 25 Это выше, чем фактическое число. Пожалуйста, попробуйте еще раз. Пожалуйста, введите число от 0 до 100: 15 Это выше, чем фактическое число. Пожалуйста, попробуйте еще раз. Пожалуйста, введите число от 0 до 100: 10 Это ниже, чем фактическое число. Пожалуйста, попробуйте еще раз. Пожалуйста, введите число от 0 до 100: 11 Это ниже, чем фактическое число. Пожалуйста, попробуйте еще раз. Пожалуйста, введите число от 0 до 100: 123 Это выше, чем фактическое число. Пожалуйста, попробуйте еще раз. Пожалуйста, введите число от 0 до 100: 12 Это правильный номер Спасибо, что играете в Number Guess. Еще увидимся
Блок - схема:

Образец раствора -2:
Вы получаете 6 шансов угадать число, которое компьютер случайно выбрал от 1 до 100.
Код Python:
# Guess a number game
#https://github.com/hardikvasa/guess-the-number-game
#Add feature to check if the input number was between 1-100
#Add levels to the game to make it more exciting
from random import randint #To generate a random number
name = input("Please Enter your name: ")
print("Welcome to my Number game, " + name)
def game():
rand_number = randint(0,100) #Generates a random number
print("\nI have selected a number between 1 to 100...")
print("You have 6 chances to guess that number...")
i = 1
r = 1
while i<7: #6 Chances to the user
user_number = int(input('Enter your number: '))
if user_number < rand_number:
print("\n" + name + ", My number is greater than your guessed number")
print("you now have " + str(6-i)+ " chances left" )
i = i+1
elif user_number > rand_number:
print("\n" + name + ", My number is less than your guessed number")
print("you now have " + str(6-i)+ " chances left" )
i = i+1
elif user_number == rand_number:
print("\nCongratulations "+name+"!! You have guessed the correct number!")
r = 0;
break
else:
print("This is an invalid number. Please try again")
print("you now have " + str(6-i)+ " chances left" )
continue
if r==1:
print("Sorry you lost the game!!")
print("My number was =" + str(rand_number))
def main():
game()
while True:
another_game = input("Do you wish to play again?(y/n): ")
if another_game =="y":
game()
else:
break
main()
print("\nEnd of the Game! Thank you for playing!")
Пример вывода:
Пожалуйста, введите ваше имя: Джон Мик Добро пожаловать в мою игру с числами, Джон Мик Я выбрал число от 1 до 100 ... У вас есть 6 шансов угадать это число ... Введите ваш номер: 15 Jhon Mick, мой номер больше вашего предполагаемого числа теперь у вас осталось 5 шансов Введите ваш номер: 12 Jhon Mick, мой номер больше вашего предполагаемого числа у вас осталось 4 шанса Введите ваш номер: 10 Jhon Mick, мой номер больше вашего предполагаемого числа теперь у вас осталось 3 шанса Введите ваш номер: 25 Jhon Mick, мой номер больше вашего предполагаемого числа у вас осталось 2 шанса Введите ваш номер: 16 Jhon Mick, мой номер больше вашего предполагаемого числа теперь у вас есть 1 шанс Введите ваш номер: 35 Jhon Mick, мой номер больше вашего предполагаемого числа теперь у вас осталось 0 шансов Извините, вы проиграли! Мой номер был = 78 Вы хотите играть снова? (Да / нет):
Блок - схема:

Пример решения -3:
Код Python:
# This is a guess the number game.
#https://github.com/InterfaithCoding/GuessNumber/blob/master/guess.py
import random
guessesTaken = 0
print('Hello! What is your name?')
myName = input()
number = random.randint(1, 20)
print('Well, ' + myName + ', I am thinking of a number between 1 and 20.')
while guessesTaken < 6:
print('Take a guess.') # There are four spaces in front of print.
guess = input()
guess = int(guess)
guessesTaken = guessesTaken + 1
if guess < number:
print('Your guess is too low.') # There are eight spaces in front of print.
if guess > number:
print('Your guess is too high.')
if guess == number:
break
if guess == number:
guessesTaken = str(guessesTaken)
print('Good job, ' + myName + '! You guessed my number in ' + guessesTaken + ' guesses!')
if guess != number:
number = str(number)
print('Nope. The number I was thinking of was ' + number)
Пример вывода:
Здравствуйте! Как тебя зовут? Джон Мик Ну, Джон Мик, я думаю о числе от 1 до 20. Предположить. 15 Ваше предположение слишком низкое. Предположить. 12 Ваше предположение слишком низкое. Предположить. 19 Ваше предположение слишком высоко. Предположить. 16 Ваше предположение слишком низкое. Предположить. 18 Ваше предположение слишком высоко. Предположить. 17 Хорошая работа, Джон Мик! Вы угадали мой номер в 6 догадках!
Блок - схема:
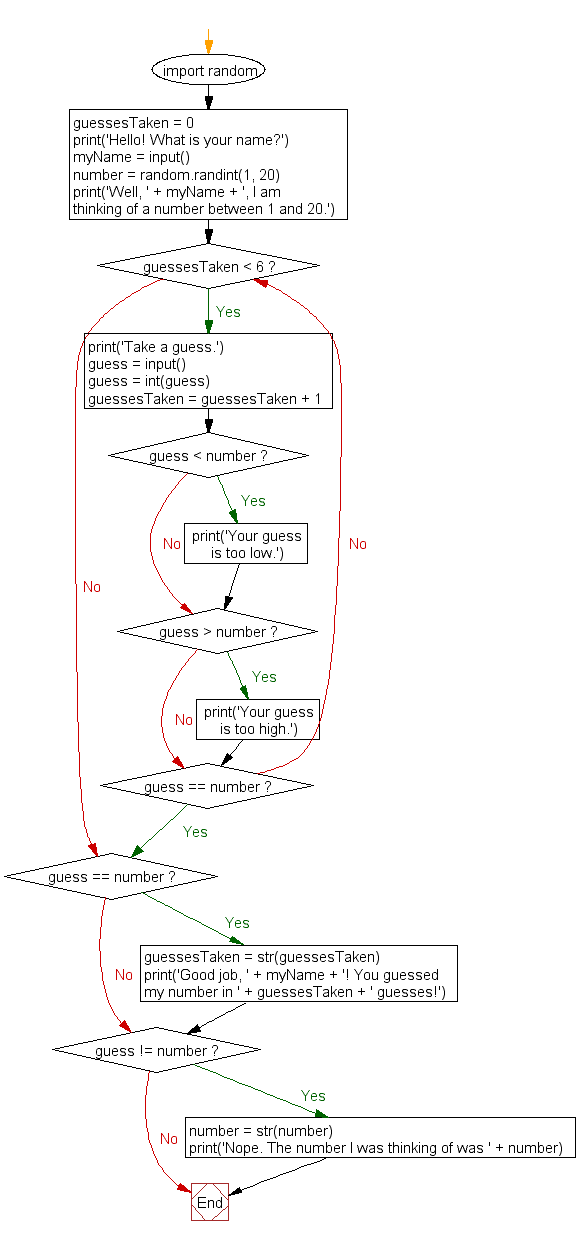
Пример решения -4:
Код Python:
#https://github.com/SomeStrHere/GuessNumber/blob/master/GuessNumber.py
import sys
from random import randint
def gameMenu() :
"""Display welcome message and initial menu to user."""
print('\nHello, welcome to Guess Number!\n')
print('Ready to play...?')
menuChoice = input('Press Y to start game, or X to quit.\n\n').upper()
menuLoop = True
while menuLoop :
clearConsole(0)
if menuChoice =="Y" :
clearConsole(0)
playGame()
elif menuChoice =="X" :
clearConsole(0)
sys.exit()
else :
gameMenu()
def playGame() :
"""Obtain input from user, check against random number and display output"""
intLower = 0 #default set to 0
intHigher = 1000 #default set to 1000
rndNumber = 0
print('\nThe number will be from an inclusive range of {0} to {1}'.format(intLower, intHigher))
try :
userGuess = int(input('Please enter your guess: '))
except :
try :
userguess = int(input('There was an error! Please enter a whole number from the range {0} - {1}\
'.format(intLower, intHigher)))
except :
print('Sorry, there was an error.')
clearConsole(2)
gameMenu()
rndNumber = randomNumber(intLower, intHigher)
while userGuess != rndNumber :
print("\nSorry, you didn't guess the number")
if userGuess < rndNumber :
print('Your guess was low')
try :
userGuess = int(input("\nWhat's your next guess? "))
except :
try :
userguess = int(input('\nSorry, there was an error; please try again: '))
except :
print('Sorry, there was an error.')
clearConsole(2)
gameMenu()
if userGuess > rndNumber :
print('Your guess was high')
try :
userGuess = int(input("What's your next guess? "))
except :
try :
userguess = int(input('\nSorry, there was an error; please try again: '))
except :
print('Sorry, there was an error.')
clearConsole(2)
gameMenu()
print('\n\nCongratulations! you guessed the number.')
print('Returning you to the menu...')
clearConsole(3)
gameMenu()
def randomNumber(a, b) :
"""Generates a random int from range a, b"""
return(randint(a,b))
def clearConsole(wait) : #function to clear console on Linux or Windows
"""Clears console, with optional time delay.
Will attempt to clear the console for Windows, should that fail it will attempt to clear the
console for Linux.
"""
import time
time.sleep(wait)
# produces a delay based on the argument given to clearConsole()
import os
try :
os.system('cls') #clears console on Windows
except :
os.system('clear') #clears console on Linux
def main() :
gameMenu()
if __name__ =="__main__" :
main()
Пример вывода:
Привет, добро пожаловать в Угадай номер! Готов играть...? Нажмите Y, чтобы начать игру, или X, чтобы выйти. Y Число будет в диапазоне от 0 до 1000 Пожалуйста, введите ваше предположение: 250 Извините, вы не угадали номер Ваше предположение было низким Каково ваше следующее предположение? 568 Извините, вы не угадали номер Ваше предположение было низким Каково ваше следующее предположение? 879 Ваше предположение было высоким Каково ваше следующее предположение? 735 Извините, вы не угадали номер Ваше предположение было высоким Каково ваше следующее предположение? 655 Извините, вы не угадали номер Ваше предположение было высоким Каково ваше следующее предположение? 539 Извините, вы не угадали номер Ваше предположение было низким Каково ваше следующее предположение? 590 Извините, вы не угадали номер Ваше предположение было низким Каково ваше следующее предположение? 600 Извините, вы не угадали номер Ваше предположение было низким Каково ваше следующее предположение? 665 Ваше предположение было высоким Каково ваше следующее предположение? 635 Извините, вы не угадали номер Ваше предположение было низким Каково ваше следующее предположение? 645 Поздравляем! ты угадал номер. Возвращая вас в меню ... Привет, добро пожаловать в Угадай номер!
Блок - схема:

Улучшите этот пример решения и опубликуйте свой код через Disqus
Новый контент: Composer: менеджер зависимостей для PHP , R программирования
disqus2code