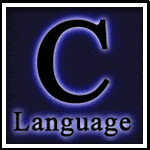
Упражнения C: Создайте односвязный список и подсчитайте количество узлов.
C связанный список: упражнение 3 с решением
Напишите программу на C, чтобы создать односвязный список из n узлов и подсчитать количество узлов.
Иллюстрированная презентация:
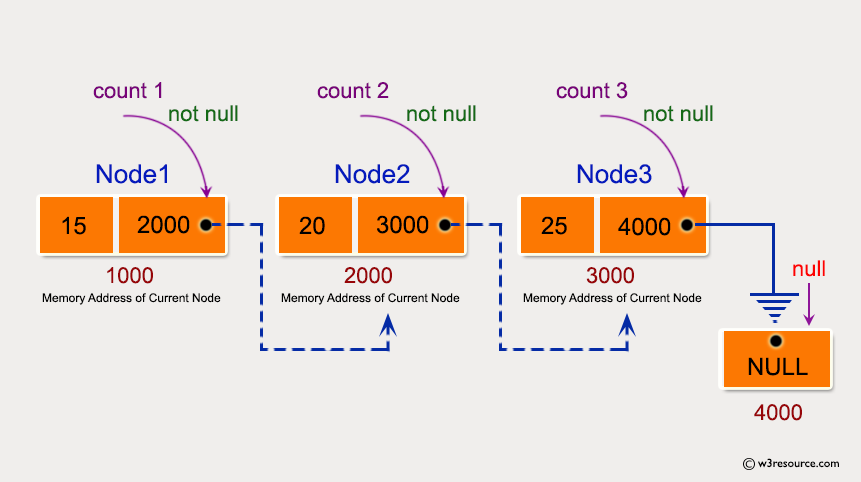
Пример решения:
Код C:
#include <stdio.h>
#include <stdlib.h>
struct node
{
int num; //Data of the node
struct node *nextptr; //Address of the node
}*stnode;
void createNodeList(int n); //function to create the list
int NodeCount(); //function to count the nodes
void displayList(); //function to display the list
int main()
{
int n,totalNode;
printf("\n\n Linked List : Create a singly linked list and count the number of nodes :\n");
printf("------------------------------------------------------------------------------\n");
printf(" Input the number of nodes : ");
scanf("%d", &n);
createNodeList(n);
printf("\n Data entered in the list are : \n");
displayList();
totalNode = NodeCount();
printf("\n Total number of nodes = %d\n", totalNode);
return 0;
}
void createNodeList(int n)
{
struct node *fnNode, *tmp;
int num, i;
stnode = (struct node *)malloc(sizeof(struct node));
if(stnode == NULL) //check whether the stnode is NULL and if so no memory allocation
{
printf(" Memory can not be allocated.");
}
else
{
// reads data for the node through keyboard
printf(" Input data for node 1 : ");
scanf("%d", &num);
stnode-> num = num;
stnode-> nextptr = NULL; //Links the address field to NULL
tmp = stnode;
//Creates n nodes and adds to linked list
for(i=2; i<=n; i++)
{
fnNode = (struct node *)malloc(sizeof(struct node));
if(fnNode == NULL) //check whether the fnnode is NULL and if so no memory allocation
{
printf(" Memory can not be allocated.");
break;
}
else
{
printf(" Input data for node %d : ", i);
scanf(" %d", &num);
fnNode->num = num; // links the num field of fnNode with num
fnNode->nextptr = NULL; // links the address field of fnNode with NULL
tmp->nextptr = fnNode; // links previous node i.e. tmp to the fnNode
tmp = tmp->nextptr;
}
}
}
}
int NodeCount()
{
int ctr = 0;
struct node *tmp;
tmp = stnode;
while(tmp != NULL)
{
ctr++;
tmp = tmp->nextptr;
}
return ctr;
}
void displayList()
{
struct node *tmp;
if(stnode == NULL)
{
printf(" No data found in the list.");
}
else
{
tmp = stnode;
while(tmp != NULL)
{
printf(" Data = %d\n", tmp->num); // prints the data of current node
tmp = tmp->nextptr; // advances the position of current node
}
}
}
Пример вывода:
Связанный список: создайте односвязный список и подсчитайте количество узлов: -------------------------------------------------- ---------------------------- Введите количество узлов: 3 Входные данные для узла 1: 5 Входные данные для узла 2: 6 Входные данные для узла 3: 7 Данные, введенные в список: Данные = 5 Данные = 6 Данные = 7 Общее количество узлов = 3
Блок - схема:
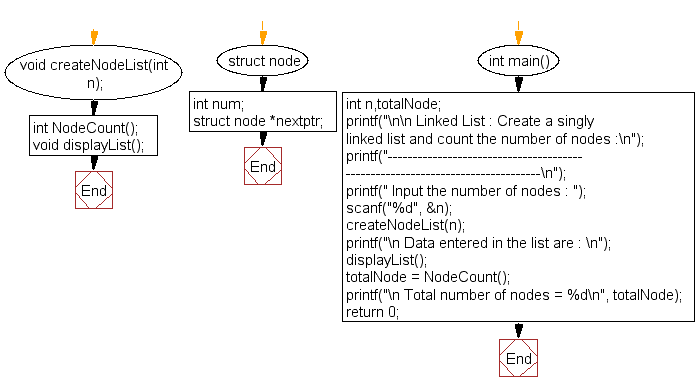
createNodeList ():
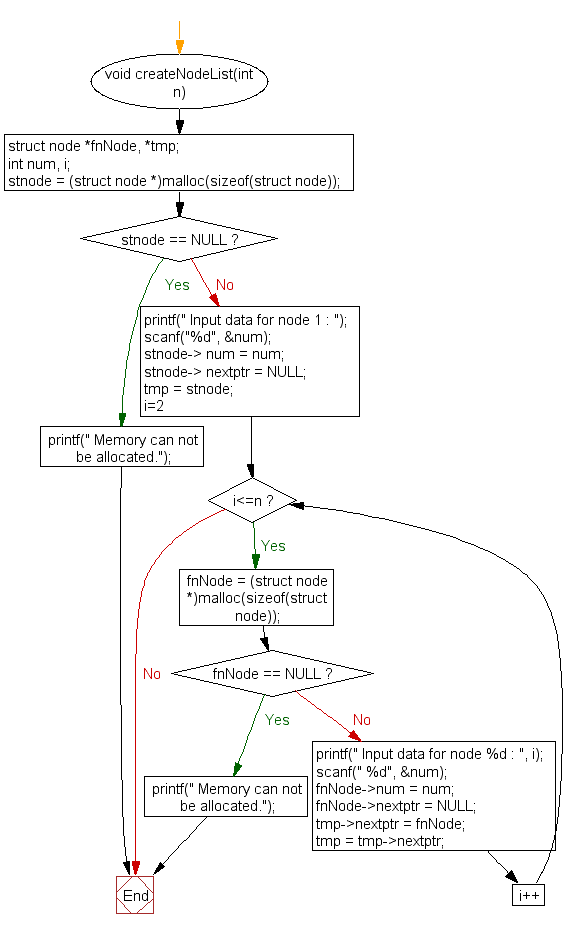
NodeCount ():
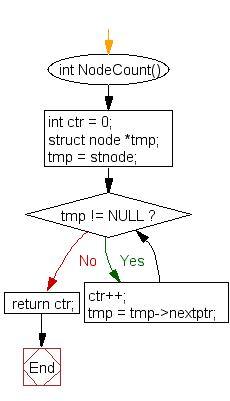
displayList ():
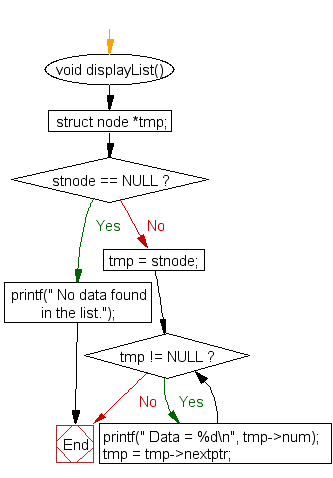
Редактор кода программирования C:
Есть другой способ решить это решение? Внесите свой код (и комментарии) через Disqus.
Предыдущий: Напишите программу на C, чтобы создать односвязный список из n узлов и отобразить его в обратном порядке.
Далее: Напишите программу на C для вставки нового узла в начало односвязного списка.
Каков уровень сложности этого упражнения?
Новый контент: Composer: менеджер зависимостей для PHP , R программирования
disqus2code